How to let React Native app and Web authentication share information about login user?
Configure Google OAuth Client to support iOS app

Configure Cognito to support Google as an authentication provider
Add Open ID Identity Provider
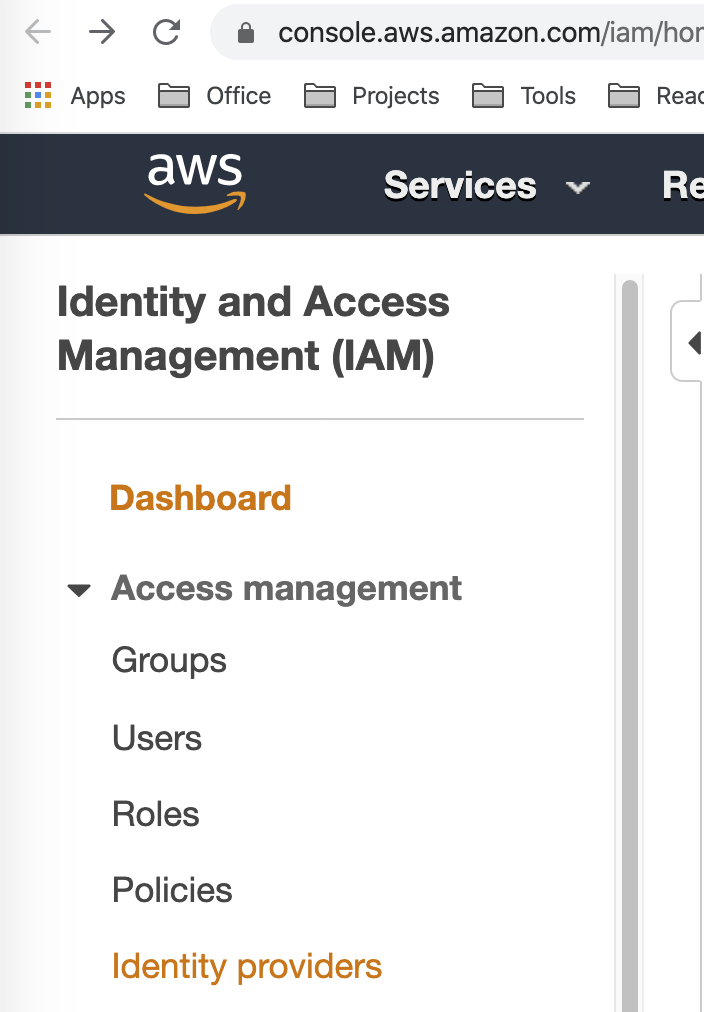
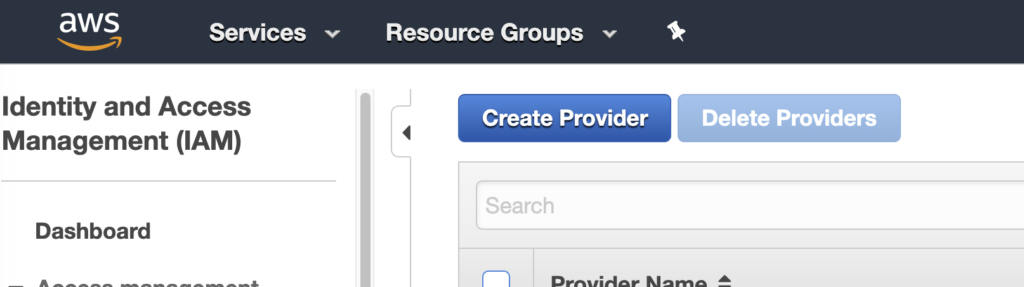
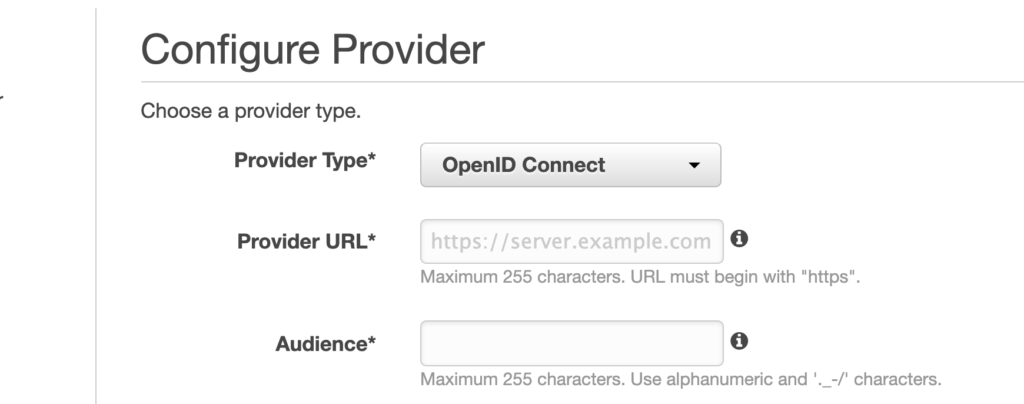
with Provider URL as “https://accounts.google.com” and Audience with Google iOS Client ID
Enable Open ID in Identity Pool
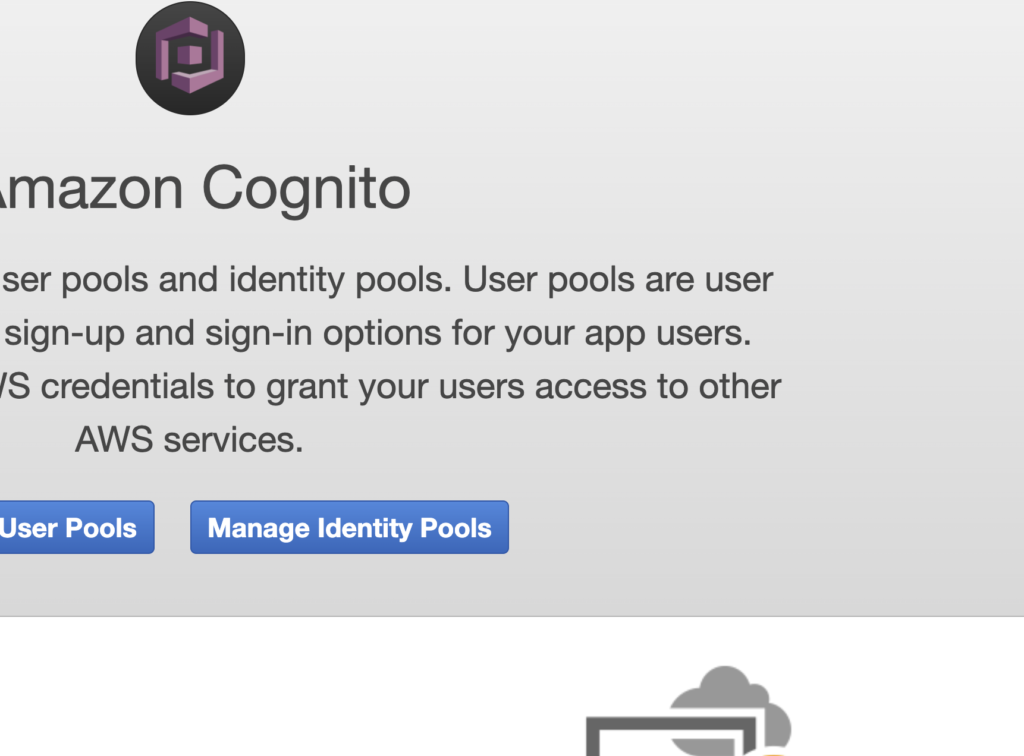
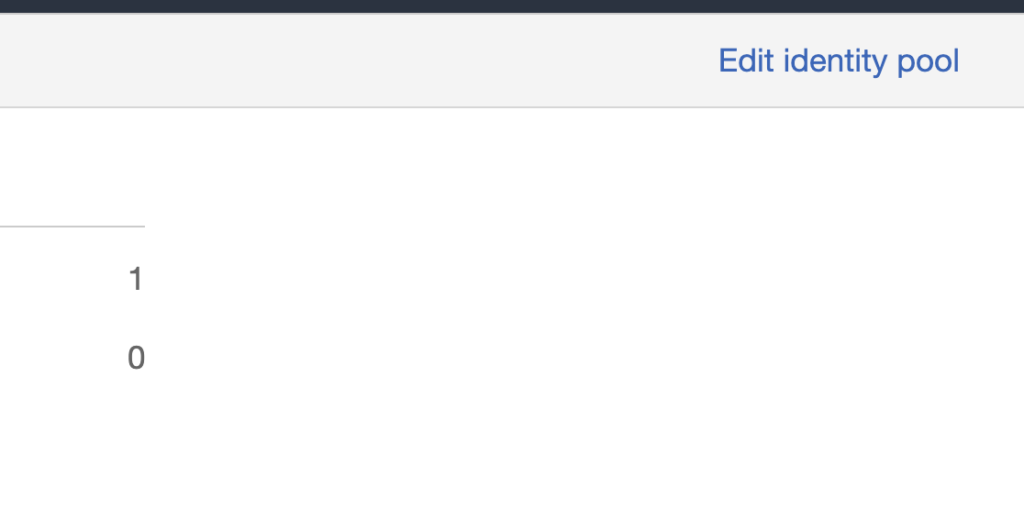
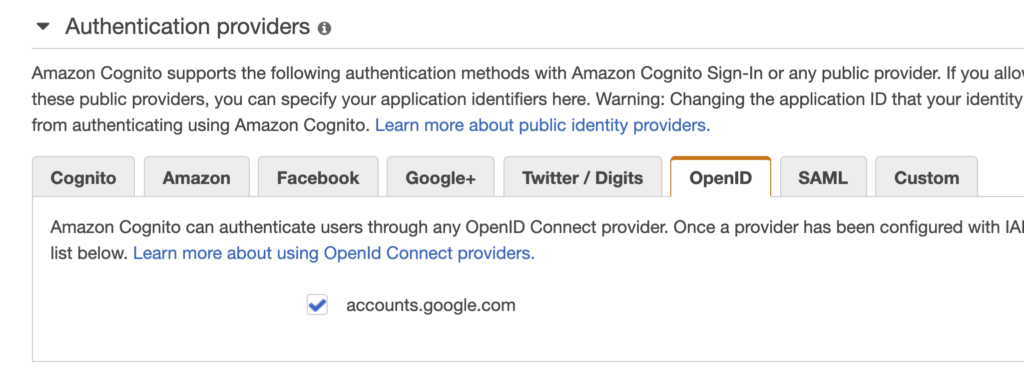
Install “react-native-google-signin”
Edit pod file and install pod
pod 'GoogleSignIn', '~> 5.0.2'
yarn add @react-native-community/google-signin
$ cd ios $ pod install
Create Google Login in React Native app
/** * Sample React Native App * https://github.com/facebook/react-native * * @format * @flow */ import React from 'react'; import { SafeAreaView, StyleSheet, ScrollView, StatusBar, Button, } from 'react-native'; import {Header, Colors} from 'react-native/Libraries/NewAppScreen'; import {Auth} from 'aws-amplify'; import Amplify from 'aws-amplify'; import config from './aws-exports'; Amplify.configure(config); import {GoogleSignin} from '@react-native-community/google-signin'; GoogleSignin.configure({ scopes: ['openid', 'email', 'profile'], webClientId: 'xxxxxxxx.apps.googleusercontent.com', offlineAccess: true, // if you want to access Google API on behalf of the user FROM YOUR SERVER hostedDomain: '', // specifies a hosted domain restriction // loginHint: '', // [iOS] The user's ID, or email address, to be prefilled in the authentication UI if possible. [See docs here](https://developers.google.com/identity/sign-in/ios/api/interface_g_i_d_sign_in.html#a0a68c7504c31ab0b728432565f6e33fd) // forceConsentPrompt: true, // [Android] if you want to show the authorization prompt at each login. // accountName: '', // [Android] specifies an account name on the device that should be used iosClientId: 'xxxxxxx.apps.googleusercontent.com', // [iOS] optional, if you want to specify the client ID of type iOS (otherwise, it is taken from GoogleService-Info.plist) }); const App: () => React$Node = () => { const signInGoogle = () => { GoogleSignin.hasPlayServices() .then(() => { GoogleSignin.signIn().then(userInfo => { console.log('userInfo', userInfo); Auth.federatedSignIn( 'google', { token: userInfo.idToken, expires_at: 60 * 1000 + new Date().getTime(), // the expiration timestamp }, userInfo.user, ) .then(cred => { // If success, you will get the AWS credentials console.log('cred', cred); return Auth.currentAuthenticatedUser(); }) .then(user => { // If success, the user object you passed in Auth.federatedSignIn console.log('user after federated login', user); }) .catch(e => { console.log('federated login error', e); }); }); }) .catch(error => { console.log('userInfo error', error); }); }; return ( <> <StatusBar barStyle="dark-content" /> <SafeAreaView> <ScrollView contentInsetAdjustmentBehavior="automatic" style={styles.scrollView}> <Header /> <Button title="Sign in with Google" onPress={() => signInGoogle()} /> </ScrollView> </SafeAreaView> </> ); }; const styles = StyleSheet.create({ scrollView: { backgroundColor: Colors.lighter, }, }); export default App;
How to create Google OAuth client for Android target?
https://developers.google.com/identity/sign-in/android/start#configure-a-google-api-project
Remember to create SHA1 from debug keystore like this
keytool -exportcert -keystore [react native path]/android/app/debug.keystore -list -v
Leave a Reply