Install “react-native-firebase”
yarn add @react-native-firebase/app
Android
- Download “google-services.json” file from Firebase Android
- There is step of generating debug signing certificate
https://round.fun/2020/05/07/android-development-setup/
- There is step of generating debug signing certificate
- Paste “google-services.json” to “android\app\” folder
- Edit “/android/build.gradle“
buildscript { dependencies { // ... other dependencies classpath 'com.google.gms:google-services:4.2.0' // Add me --- /\ } }
- Edit “/android/app/build.gradle“
apply plugin: 'com.android.application' apply plugin: 'com.google.gms.google-services' // <- Add this line
iOS
- Download “GoogleService-Info.plist” file from Firebase iOS
- Open iOS app from XCode, add “GoogleService-Info.plist” into project
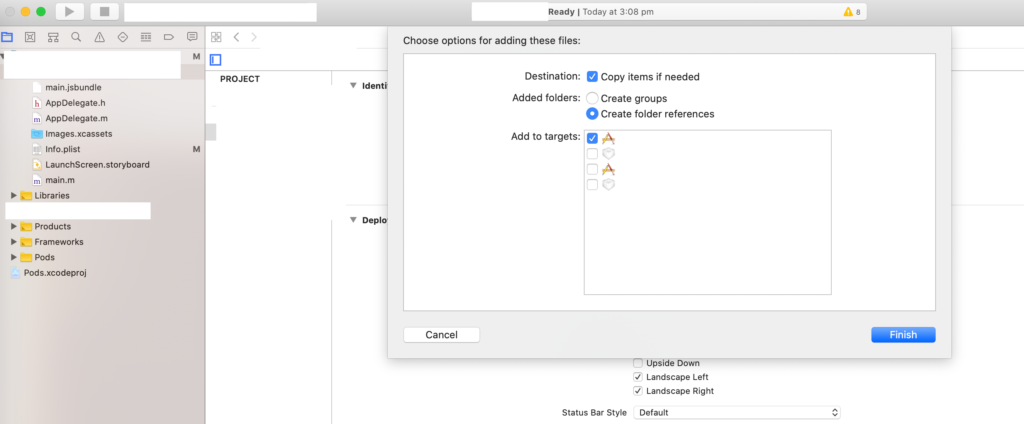
- Fix AppDelegate.m
https://rnfirebase.io/#configure-firebase-with-ios-credentials
Autolinking & rebuilding
npx react-native run-android # iOS apps cd ios/ pod install --repo-update cd .. npx react-native run-ios
Install FCM
yarn add @react-native-firebase/messaging
Configure iOS app
- Turn on capabilities
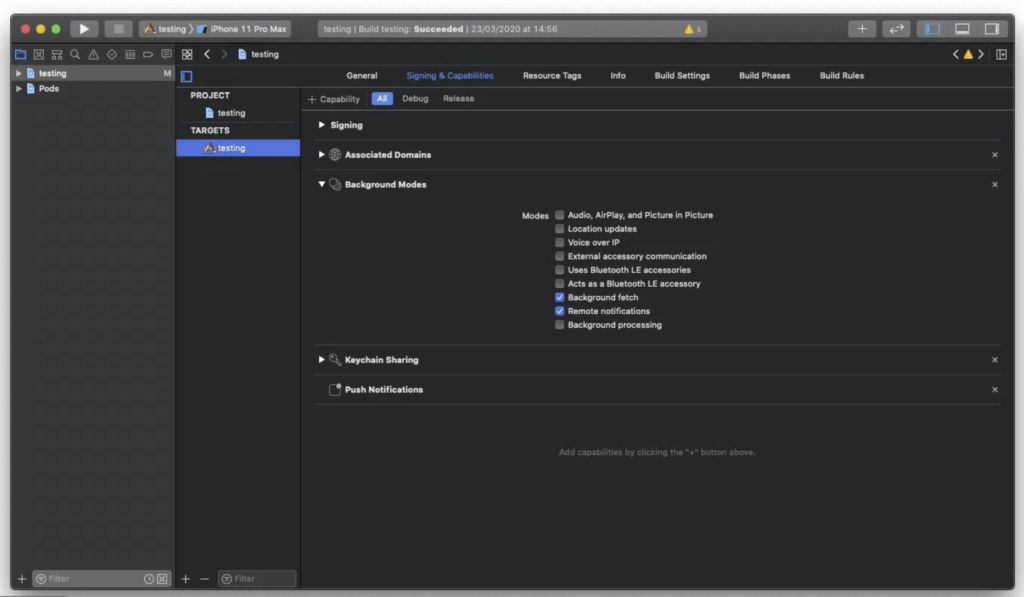
- Create p8 file and upload p8 file to Firebase
https://round.fun/2020/08/01/mobile-app-how-to-configure-push-notification-with-firebase/ - Create push notification certificate
https://round.fun/2020/08/01/mobile-app-how-to-configure-push-notification-with-firebase/
Create FCM class
import messaging from '@react-native-firebase/messaging'; export class FCMService { // Check permission async checkPermission() { const enabled = await messaging().hasPermission(); // If Permission granted proceed towards token fetch if (enabled) { this.getToken(); } else { // If permission hasn’t been granted to our app, request user in requestPermission method. this.requestPermission(); } } // Request permission async requestPermission() { const authStatus = await messaging().requestPermission(); const enabled = authStatus === messaging.AuthorizationStatus.AUTHORIZED || authStatus === messaging.AuthorizationStatus.PROVISIONAL; if (enabled) { this.getToken(); } } // Get device token async getToken() { try { const fcmToken = await messaging().getToken(); if (fcmToken) { // this.saveTokenToDatabase(fcmToken); } } catch (error) { console.log('FCM Service: User does not have a device token', error); } } // Refresh device token async refreshToken() { messaging().onTokenRefresh((token) => { // this.saveTokenToDatabase(token); }); } // Listen notification tapped async createNotificationListeners() { // This listener triggered when notification has been received in foreground messaging().onMessage(async (remoteMessage) => { console.log('A new FCM message arrived!', remoteMessage); }); // This listener triggered when app is in background and we click, tapped and opened notification messaging().onNotificationOpenedApp((remoteMessage) => { console.log( 'Notification caused app to open from background state:', remoteMessage.notification ); }); // This listener triggered when app is closed and we click,tapped and opened notification messaging() .getInitialNotification() .then((remoteMessage) => { if (remoteMessage) { console.log( 'Notification caused app to open from quit state:', remoteMessage.notification ); } }); } }
Call FCM class
import { FCMService } from './source/_services/fcmService'; const fcmService = new FCMService(); const App = () => { useEffect(() => { fcmService.checkPermission(); fcmService.createNotificationListeners(); }, []); return <></> }; export default App;
Test FCM from Firebase
- You can see notification alerts when app is in background or closed
- No notification alert is displayed when app is in foreground, this is stated here
If theRemoteMessage
payload contains anotification
property when sent to theonMessage
handler, the device will not show any notification to the user. Instead, you could trigger a local notification or update the in-app UI to signal a new notification.
https://rnfirebase.io/messaging/usage#foreground-state-messages
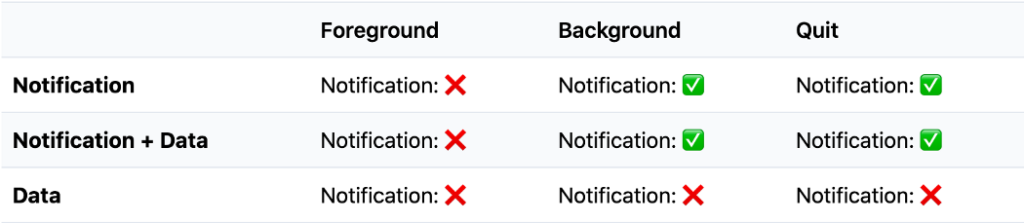
Configure background handler
When the application is in a background or quit state, the onMessage
handler will not be called when receiving messages. Instead, you need to setup a background callback handler via the setBackgroundMessageHandler
method.
https://rnfirebase.io/messaging/usage#background–quit-state-messages
Leave a Reply