How to create a page with header, content and footer?
header – main – footer
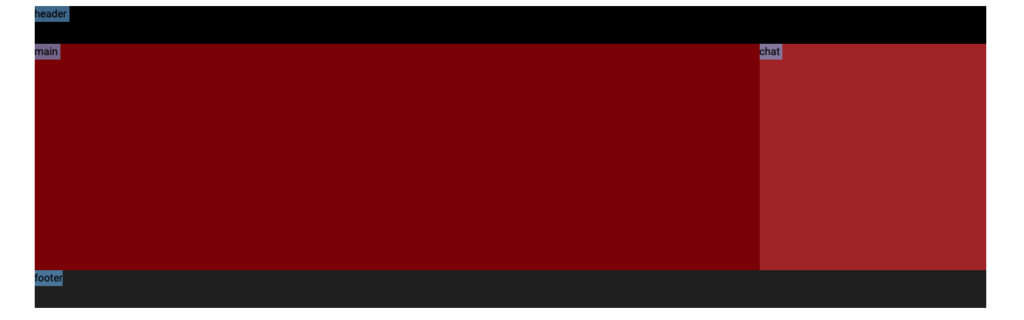
.body { display: grid; /* 2 columns */ grid-template-columns: 9fr auto; /* 3 rows */ grid-template-rows: 50px auto 50px; /* row configuration */ grid-template-areas: /* Full width of "header" area */ "header header" /* 1st column is "main" area, 2nd column is "chat" area */ "main chat" /* Full width of "footer" area */ "footer footer"; } .header{ grid-area: header; background-color: black; } .main { grid-area: main; height: 300px; background-color: maroon; } .chat{ grid-area: chat; background-color: brown; overflow: auto; width: 300px; transition: all 1s; } .body:hover .chat { width: 0px; } .footer{ grid-area: footer; background-color: #222; }
import styles from './index.module.css' ... return ( <div className={styles.body}> <div className={styles.header}>header</div> <div className={styles.main}>main</div> <div className={styles.chat}>chat</div> <div className={styles.footer}>footer</div> </div> )
How to create a table?
14 columns with different size, 15px gap between rows
<div style={{ display: 'grid', gridTemplateColumns: '25px 5fr repeat(7, 1fr) 60px', gridRowGap: '15px' }} > {children} </div>
colspan
<!-- Header --> <div style={{ display: 'grid', gridTemplateColumns: '25px 60px 5fr repeat(7, 1fr) 60px', gridRowGap: '15px' }} > <!-- 1st column: Margin left 25px --> <div></div> <!-- 2nd column: Start on column 2 and span 2 columns (60px col and 5fr col)--> <div style={{ gridColumn: '2 / span 2'}}></div> ... </div> <!-- Body --> <div style={{ display: 'grid', gridTemplateColumns: '25px 60px 5fr repeat(7, 1fr) 60px', gridRowGap: '15px' }} > <!-- 1st column: Margin left 25px --> <div></div> <!-- 2nd column: 60px --> <div> ... </div> <!-- 3rd column: 5fr --> <div> ... </div> ... </div>
Leave a Reply