How to debug a device over wifi?
- Connect device on same wifi network
- Connect device with USB
$ adb devices
- Restart TCP Mode
$ adb tcpip 5555
- Find IP address from mobile device
Settings -> About phone/tablet -> Status -> IP address - Connect device to Computer
$ adb connect 192.168.0.102
- Remove USB cable, DONE!
- Disconnect
$ adb disconnect 192.168.0.102
How to Transfer File from Android device to Mac?
- Disable “Debug” mode
- Search “Use USB for” > Select “File transfer/ Android Auto”
How to set sdk path?
- Create “local.properties” file
sdk.dir=/Users/your_name/Library/Android/sdk
How to generate debug signing certificate (ie for Firebase)?
- Go to https://developers.google.com/android/guides/client-auth
- Run
keytool -list -v -alias androiddebugkey -keystore ${debug.keystore file path}
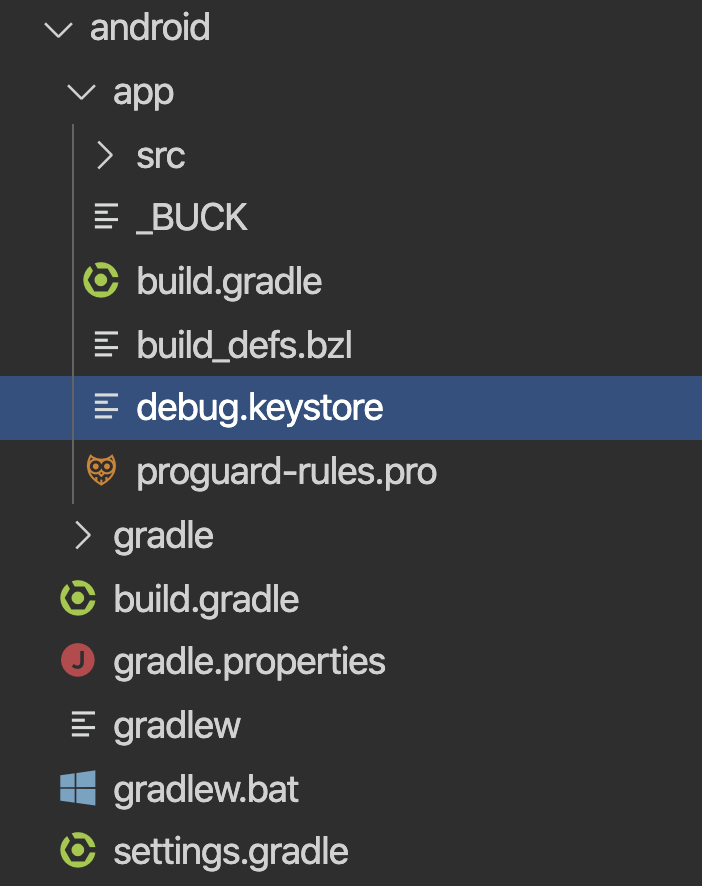
- Don’t input password, here is SHA1
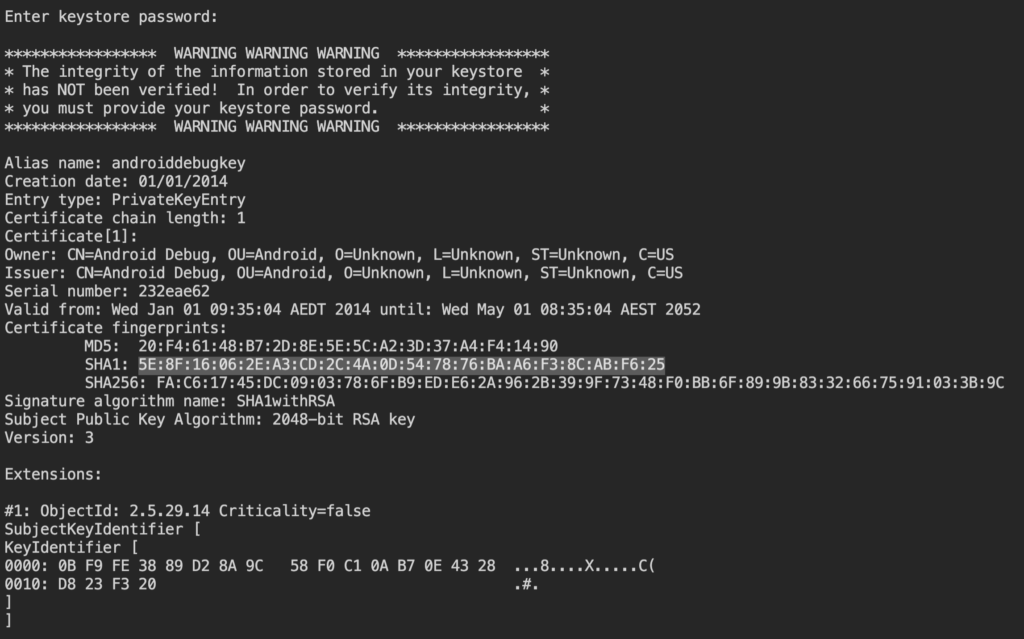
How to get SHA-256 from keystore?
Option 1: Google Play Console
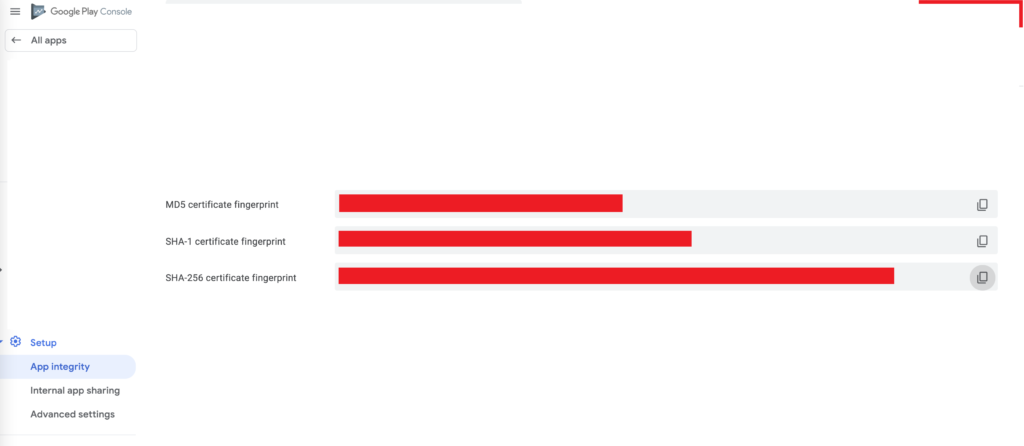
Option 2: Command line
keytool -list -v -keystore <keystore path> -alias <key alias> -storepass <store password> -keypass <key password>
How to launch an emulator in the tool window?
Android Studio > Preferences > Tools > Emulator and select/ deselect Launch in a tool window.
How to add a private SSL certificate to an Android project?
- Add pem file into src/main/res/raw/abc.pem
- Add src/main/res/xml/network_security_config.xml
<?xml version="1.0" encoding="utf-8"> <network-security-config> <domain-config> <domain includeSubdomains="true">xxxxxx.com</domain> <trust-anchors> <certificates src="@raw/abc.pem" /> </trust-anchors> </domain-config> </network-security-config>
- Add this prop into application tag in Manifest file
<application ... android:networkSecurityConfig="@xml/network_security_config" ...
How to set signing for Build variants?
- Select “Project Structure“
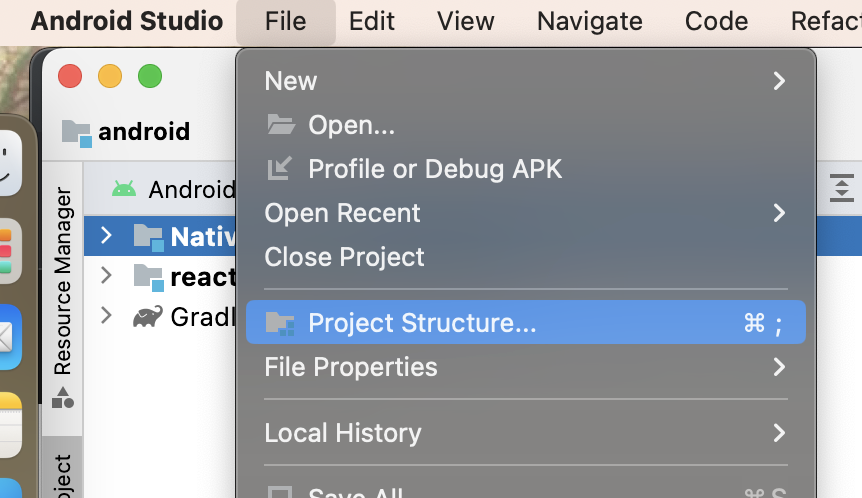
- Create a signing
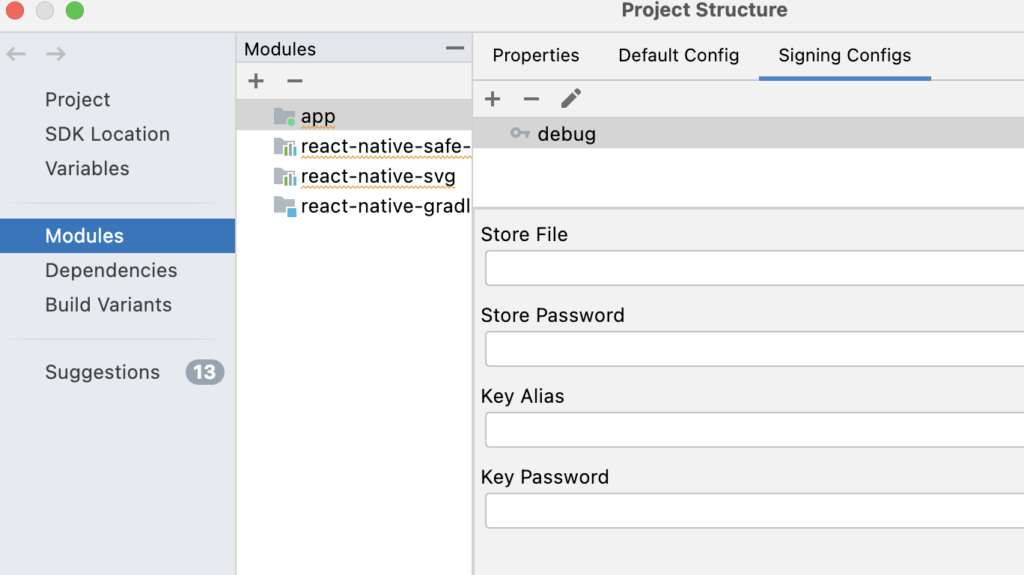
- Set this signing to a build flavour
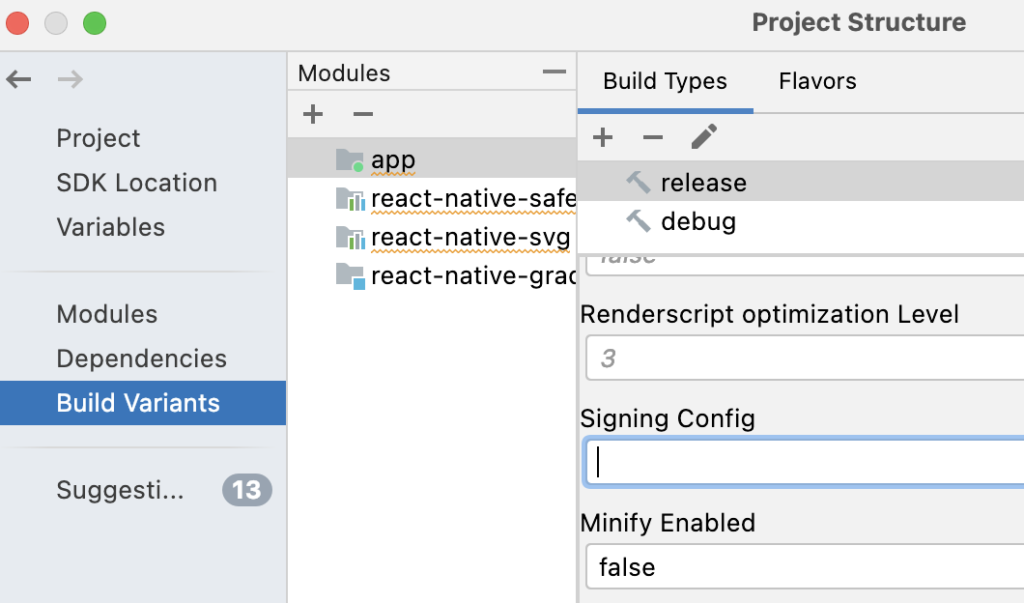
- How to build an variant by CLI?
$ gradlew tasks $ ./gradlew task-name
How to access Android emulator package?
adb shell run-as your.app.package.name ls /data/data/your.app.package.name
adb -e shell run-as your.app.package.name
How to use cli to control the emulator?
adb shell touch /sdcard/Download/xxxxx adb reboot
How to clean up Gradle?
- Remove the Gradle User Home text field content
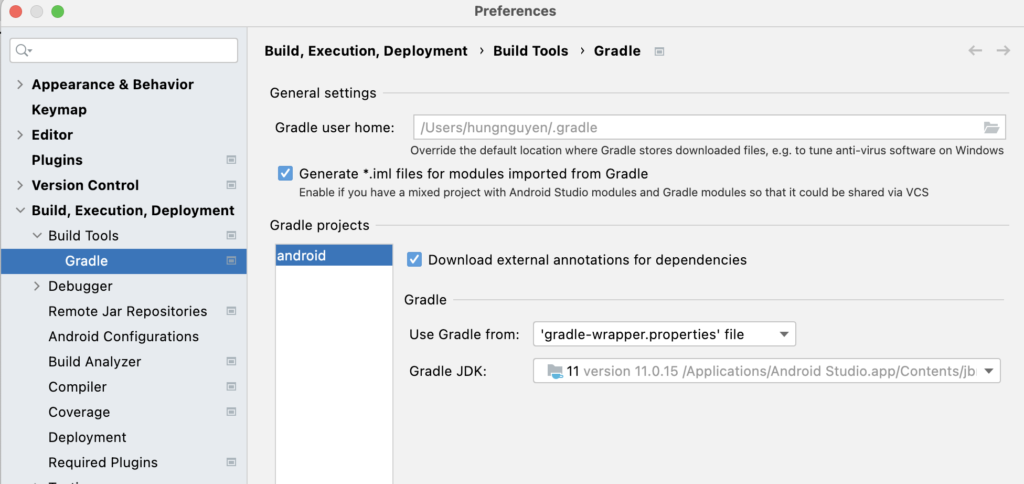
- Android Studio shows a “Reset” button
- Click “Reset”, then “OK”
Issues
How to set or change the default Java (JDK) version on macOS?
(When running ./gradlew publishToMavenLocal, it requires JDK 11+)
- Download a version of JDK you want and install it
installation-jdk-macos
- Open ~/.zshrc and set JAVA_HOME with the version you have just installed
export JAVA_HOME=$(/usr/libexec/java_home -v 16.0.2)
source ~/.zshrc echo $JAVA_HOME java -version
Android Studio Error “Android Gradle plugin requires Java 11 to run. You are currently using Java 1.8”
- Check your JVM version
./gradlew --version
- Download your new JDK as above
How to know JVM versions installed in my machine?
/usr/libexec/java_home -V
React native Android build failed after updating JVM from 1.8.0 to 16.0.2, how to fix?
- Open ~/.zshrc and set JAVA_HOME with the version 1.8.0
export JAVA_HOME=$(/usr/libexec/java_home -v 1.8.0)
Unable to load script. Make sure you are either running a Metro server or that your bundle ‘index.android.bundle’ is packaged correctly for release.
Run
adb reverse tcp:8081 tcp:8081
because
When the RN packager is running, there is an active web server accessible in your browser at 127.0.0.1:8081. It’s from this server that the JS bundle for your application is served and refreshed as you make changes. Without the reverse proxy, your phone wouldn’t be able to connect to that address.
Leave a Reply