What’s device coordinate frame?
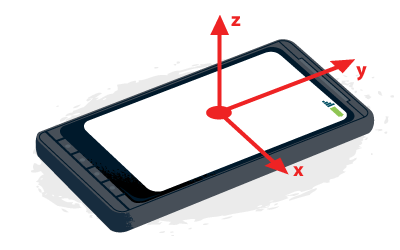
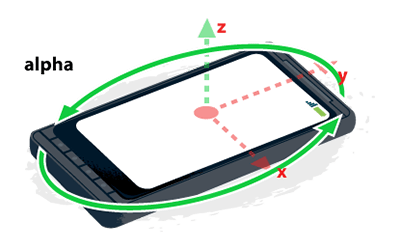
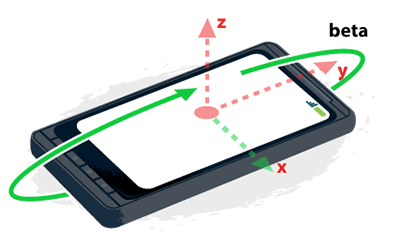
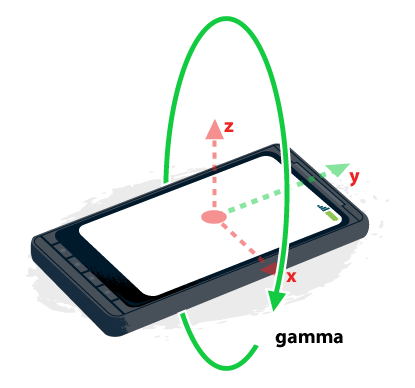
react-native-sensors
Detect device shake
import { accelerometer, setUpdateIntervalForType, SensorTypes, } from 'react-native-sensors'; import {map, filter} from 'rxjs/operators'; ... const [lastShakeTime, setLastShakeTime] = useState(0); useEffect(() => { const SHAKE_THRESHOLD = 10; // This is shake sensitivity - lowering this will give high sensitivity and increasing this will give lower sensitivity const MIN_TIME_BETWEEN_SHAKES_MILLISECS = 1000; setUpdateIntervalForType(SensorTypes.accelerometer, 200); const subscription = accelerometer .pipe( map( ({x, y, z}) => Math.sqrt( Math.pow(x, 2) + Math.pow(y, 2) + Math.pow(z, 2), ) /* - SensorManager.GRAVITY_EARTH */, ), filter((acceleration) => acceleration > SHAKE_THRESHOLD), ) .subscribe((acceleration) => { const curTime = new Date().getTime(); if (curTime - lastShakeTime > MIN_TIME_BETWEEN_SHAKES_MILLISECS) { setLastShakeTime(curTime); console.log('Shaked device! acceleration: ', acceleration); } }); return () => { subscription.unsubscribe(); }; });
But build is error in iOS !!
expo-sensors
Installation
- Install “react-native-unimodules” installing-unimodules
- Install “expo-sensors” existing-apps
yarn add expo-sensors
npx pod-install
Detect device shake
import { Accelerometer } from 'expo-sensors'; ... useEffect(() => { addSensorListener(() => { //add your code here console.log('Shake Shake Shake'); }); return () => { removeListener(); }; }); const addSensorListener = (handler) => { //this is shake sensitivity - lowering this will give high sensitivity and increasing this will give lower sensitivity const THRESHOLD = 1; let last_x, last_y, last_z; let lastUpdate = 0; Accelerometer.addListener((accelerometerData) => { let { x, y, z } = accelerometerData; let currTime = Date.now(); if (currTime - lastUpdate > 100) { let diffTime = currTime - lastUpdate; lastUpdate = currTime; let speed = (Math.abs(x + y + z - last_x - last_y - last_z) / diffTime) * 10000; if (speed > THRESHOLD) { handler(); } last_x = x; last_y = y; last_z = z; } }); }; const removeListener = () => { Accelerometer.removeAllListeners(); };
Leave a Reply