how-to-get-https-working-on-your-local-development-environment
Generate root SSL certificate
This root certificate can then be used to sign any number of certificates you might generate for individual domains
- Generate key file
openssl genrsa -des3 -out rootCA.key 2048
- Generate certificate file
openssl req -x509 -new -nodes -key rootCA.key -sha256 -days 1024 -out rootCA.pem
This certificate will have a validity of 1,024 days
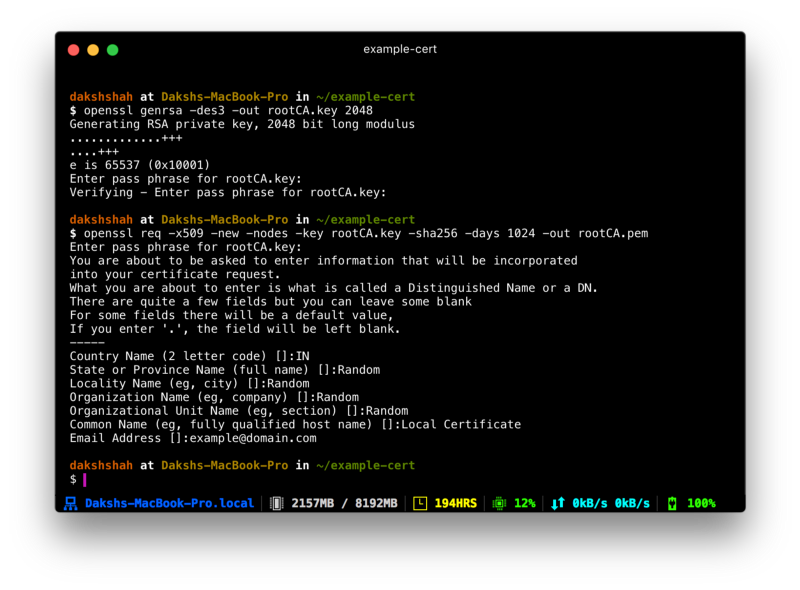
Trust SSL certificate
You need to to tell your Mac to trust your root certificate so all individual certificates issued by it are also trusted
- Double click “rootCA.pem” file to import it to Keychain Access
- Open Keychain, double click certificate you have just imported, change “When using this certificate” to “Always Trust”
Issue a domain SSL certificate for “localhost”
- Create a new OpenSSL configuration file “
server.csr.cnf"
so you can import these settings when creating a certificate instead of entering them on the command line
[req] default_bits = 2048 prompt = no default_md = sha256 distinguished_name = dn [dn] C=RD ST=random L=random O=random OU=random emailAddress=random@random.com CN = localhost
- Create a
"v3.ext"
file in order to create a X509 v3 certificate
authorityKeyIdentifier=keyid,issuer basicConstraints=CA:FALSE keyUsage = digitalSignature, nonRepudiation, keyEncipherment, dataEncipherment subjectAltName = @alt_names [alt_names] DNS.1 = localhost
- Create certificate key
openssl req -new -sha256 -nodes -out server.csr -newkey rsa:2048 -keyout server.key -config <( cat server.csr.cnf ) openssl x509 -req -in server.csr -CA rootCA.pem -CAkey rootCA.key -CAcreateserial -out server.crt -days 500 -sha256 -extfile v3.ext
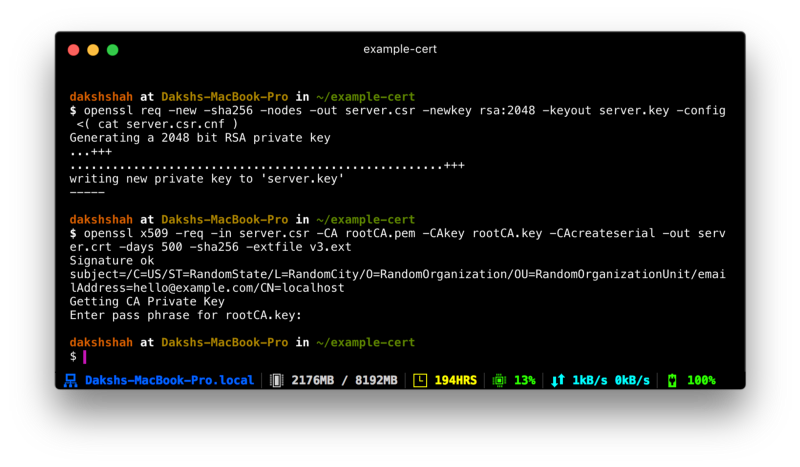
Use your new SSL certificate
Move server.key
and server.crt
to an accessible location on your server and include them when starting it.
In an Express app running on Node.js, you’d do something like this:
const path = require("path"); const fs = require("fs"); const https = require("https"); const express = require("express"); const bodyParser = require("body-parser"); const app = express(); const port = 3000; ////////////////// // Setup server app.use(bodyParser.json()); app.use( bodyParser.urlencoded({ extended: true, }) ); const certOptions = { key: fs.readFileSync(path.resolve("./server.key")), cert: fs.readFileSync(path.resolve("./server.crt")), }; const server = https.createServer(certOptions, app); // Listen request app.get("/", (request, response) => { response.json({ info: "Node.js, Express, and Postgres API" }); }); server.listen(port, () => { console.log(`App running on port ${port}.`); });
Leave a Reply