React Native – Setup config file
You need AWS side:
- Identity Pool Id
- User Pool Id
- User Pool Client Id
Install dependencies
yarn add @aws-amplify/core @aws-amplify/auth
Create “aws-exports.js“
const awsmobile = { identityPoolId: AWS_IDENTITY_POOL_ID, region: AWS_REGION, userPoolId: AWS_USER_POOL_ID, userPoolWebClientId: AWS_USER_POOL_CLIENT_ID, oauth: { // Domain name domain: AWS_DOMAIN_NAME, // Authorized scopes scope: [ 'phone', 'email', 'profile', 'openid', 'aws.cognito.signin.user.admin', ], // Callback URL redirectSignIn: AWS_REDIRECT_SIGNIN, // Sign out URL redirectSignOut: AWS_REDIRECT_SIGNOUT, // 'code' for Authorization code grant, // 'token' for Implicit grant // Note that REFRESH token will only be generated when the responseType is code responseType: 'code', }, }; export default awsmobile;
- userPoolWebClientId
There may have multiple App client id, select correct one
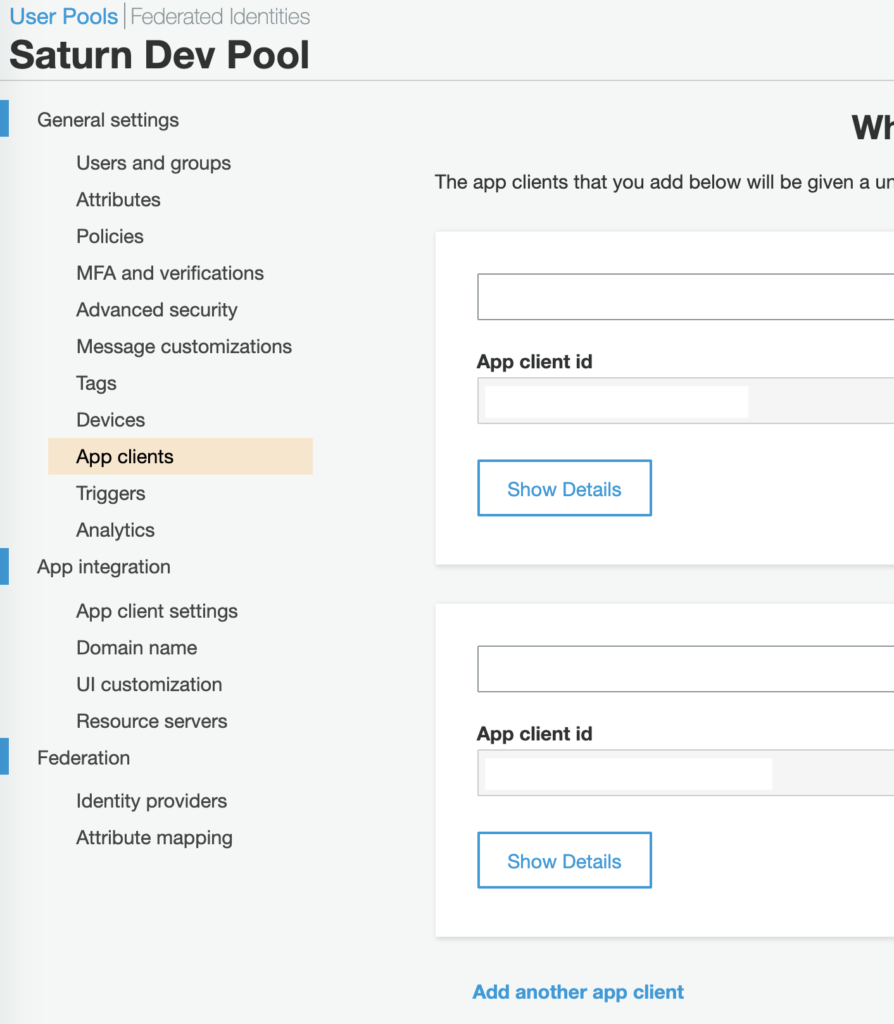
- oauth > domain
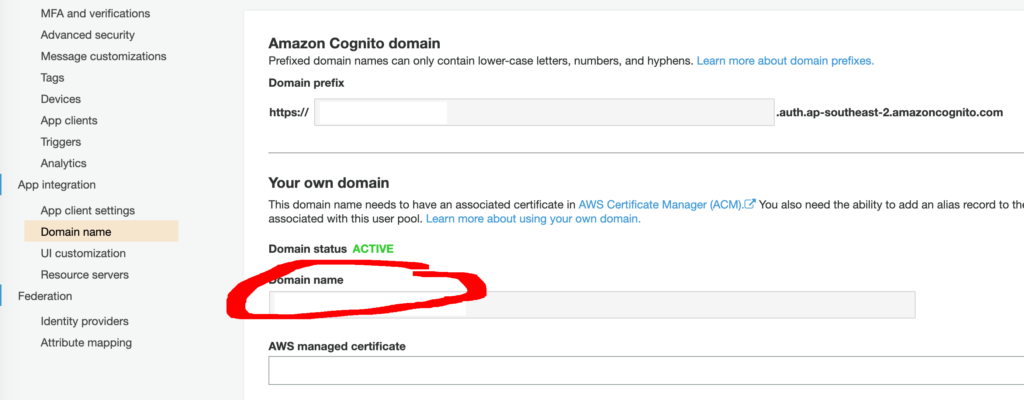
- oauth > redirectSignIn, redirectSignOut
- This is your custom URL scheme configured for your mobile app (for example: “myapp://”)
- This (for example: “myapp://”) must be configured in Callback URL(s) and Sign out URL(s) too
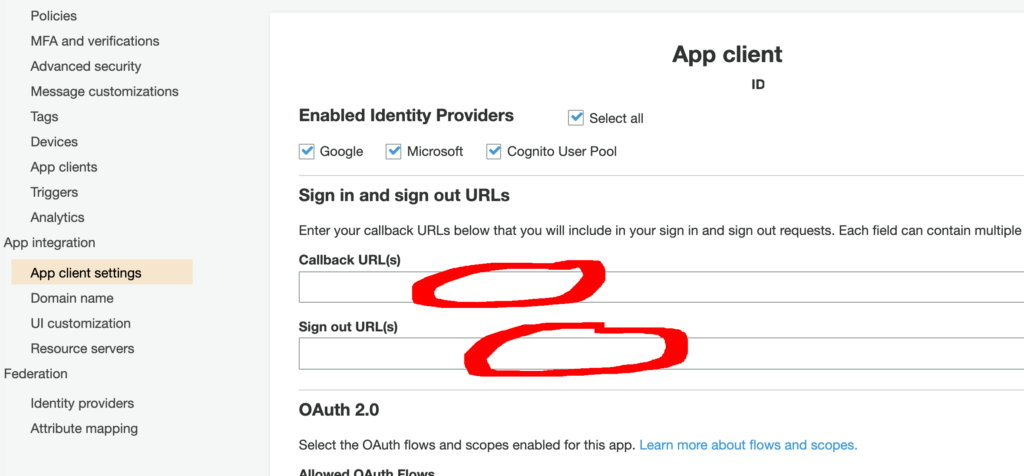
React Native – Authentication codes
- Configure Amplify at top Component
import config from 'src/config/aws-exports'; import Amplify from '@aws-amplify/core'; Amplify.configure(config);
- Configure custom URL scheme for Android in AndroidManifest.xml
<intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:scheme="myapp" /> </intent-filter>
- Configure custom URL scheme for iOS in info.plist
<key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleTypeRole</key> <string>Editor</string> <key>CFBundleURLName</key> <string>myapp</string> <key>CFBundleURLSchemes</key> <array> <string>myapp</string> </array> </dict> </array>
- Edit AppDelegate.m in iOS to make deep linking work
// iOS 9.x or newer #import <React/RCTLinkingManager.h> ... @implementation AppDelegate ... - (BOOL)application:(UIApplication *)application openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options { return [RCTLinkingManager application:application openURL:url options:options]; }
- Authentication code
import Auth from '@aws-amplify/auth'; import { Hub } from '@aws-amplify/core'; Hub.listen('auth', (data) => { const { payload } = data; console.log(payload); }); const LoginScreen = () => { const onLogin = async () => { try { await Auth.federatedSignIn(); } catch (error) { console.log(error); } }; return ( <StyledContainer> <Button title="Login" onPress={() => onLogin()} /> </StyledContainer> ); }; export default LoginScreen;
Errors
Unable to verify secret hash for client
This is because App Client you select uses secret key. Javascript can’t support this Client app, you need to recreate new App Client without generating secret key
Token is not from a supported provider of this identity pool
Check if User Pool id is set correctly in Identity Pool
Cognito Console > Federated Identities > Edit identity pool > Cognito > App client id
Invalid identity pool configuration. Check assigned IAM roles for this pool.
- You have to configure Identity roles here
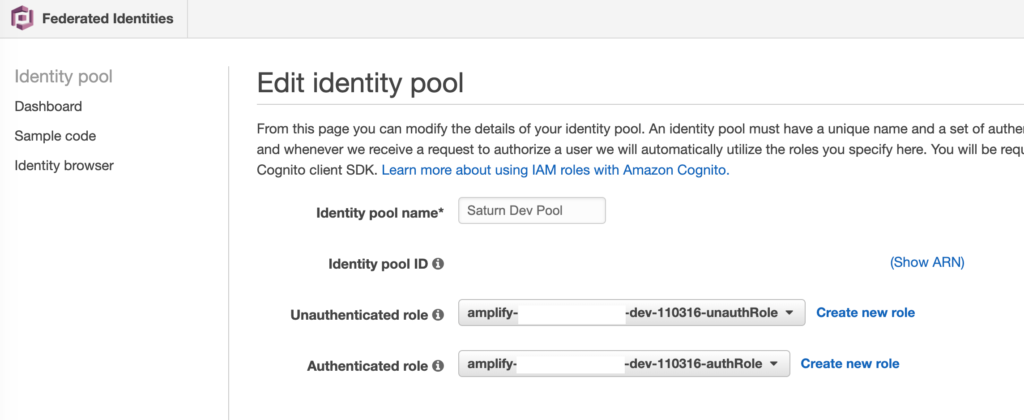
- If it’s still error, check role’s “Trust Relationship“
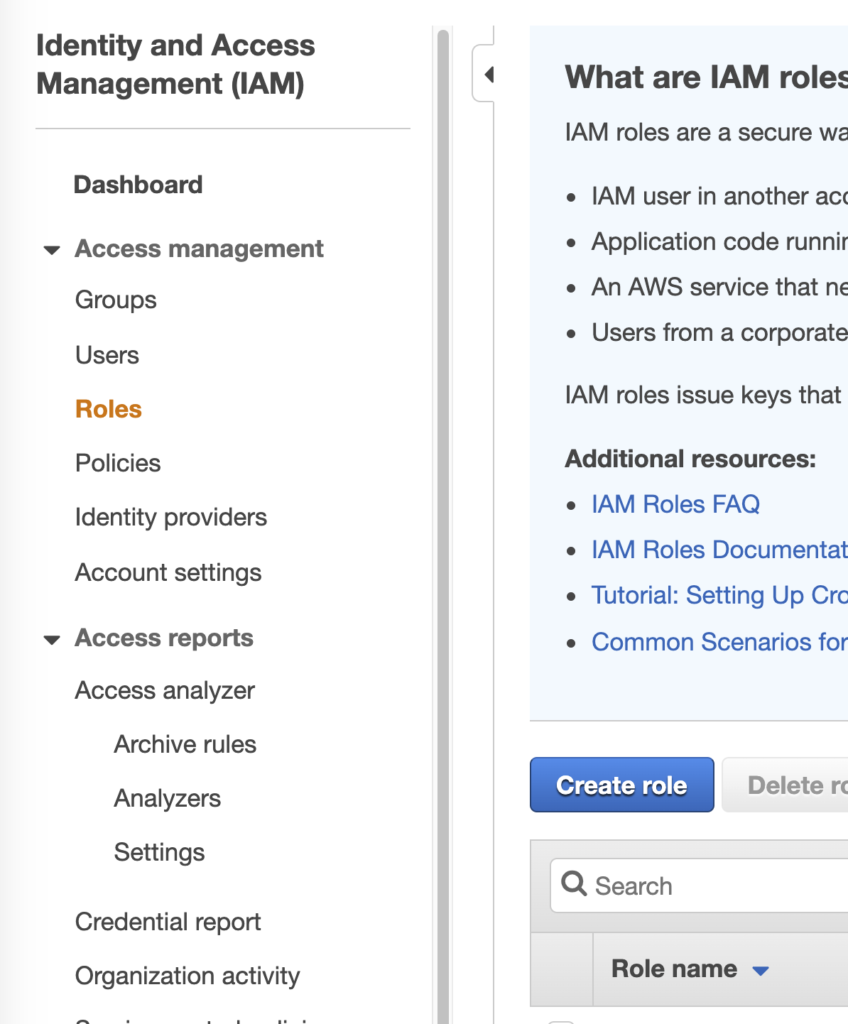
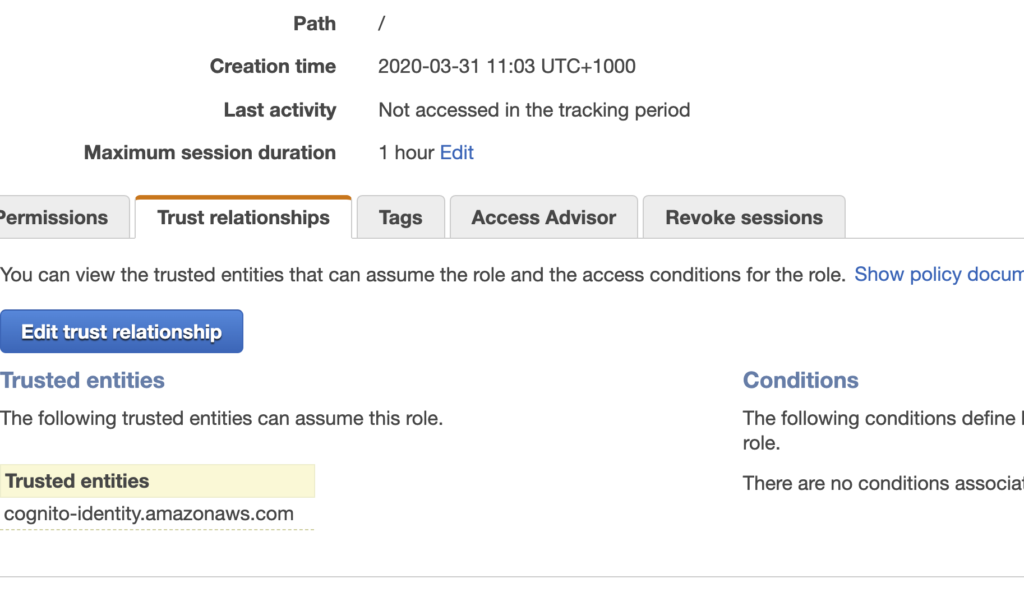
- Edit Trust Relationship
- For example: Change Effect from “Deny” to “Allow” for “AuthRole”
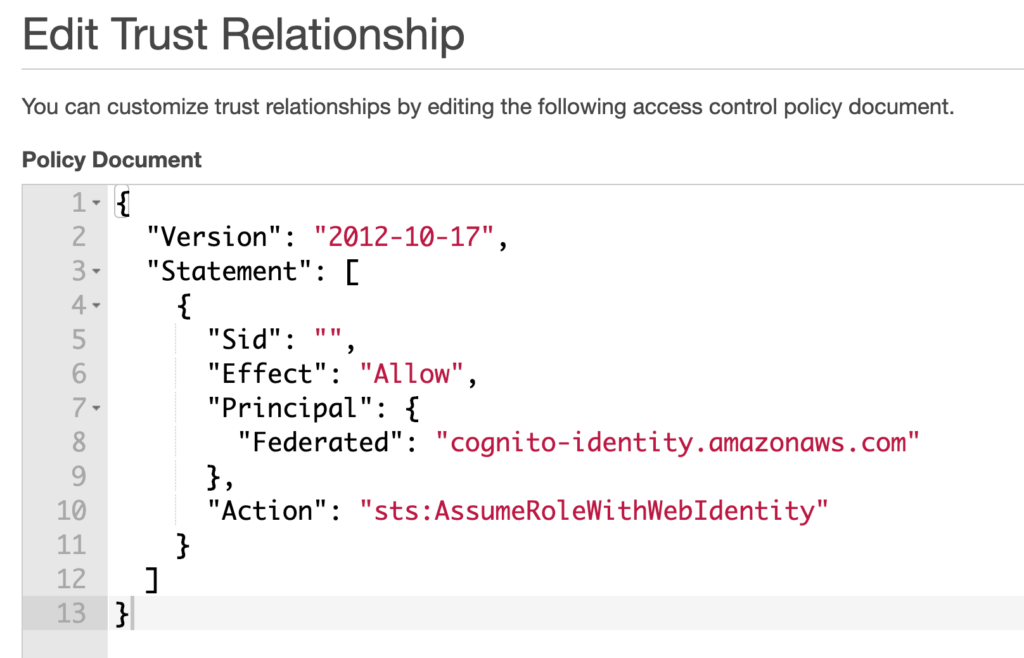
Leave a Reply