Assuming you have a custom font icon themeA.ttf file
react-native side
- Generate a fontConfig.json file
{"name":"themeA","icons":{"Icon-chev-left":"%uE1AF%uED8C%uE06B","icon- chev-right":"%uEC62%uE6A4%uE002"}}
- Create a list of icon names
// iconList.ts export enum IconName { ICON_CHEV_LEFT = 'Icon-chev-left', ICON_CHEV_RIGHT = 'icon- chev-right' }
- Create an Icon component
// Icon.tsx import React from 'react'; import styled from 'styled-components'; import IconConfig from './fontConfig.json'; import { Text } from 'react-native'; const StyledText = styled(Text).attrs({})` font-family: themeA; // Set font family here!!! color: ${(props) => (props.color ? props.color : props.theme.color.white)}; font-size: ${(props) => (props.color ? props.size : 14)}px; font-weight: ${(props) => (props.weight ? props.weight : 400)}; `; interface Props { name: string; color: string; size: number; weight?: number; } const Icon: React.FC<Props> = ({ name, color, size, weight = 400 }) => { return ( <StyledText color={color} size={size} weight={weight}> {unescape(IconConfig.icons[name])} </StyledText> ); }; export default Icon;
- Usage
import { IconName } from '../Icon/iconList'; ... <Icon name={IconName.ICON_CHEV_LEFT} color={'white'} size={25} />
Android side
Copy themeA.ttf into app/src/main/assets/fonts/themeA.ttf
iOS side
- Drop/drag themeA.ttf into Fonts folder
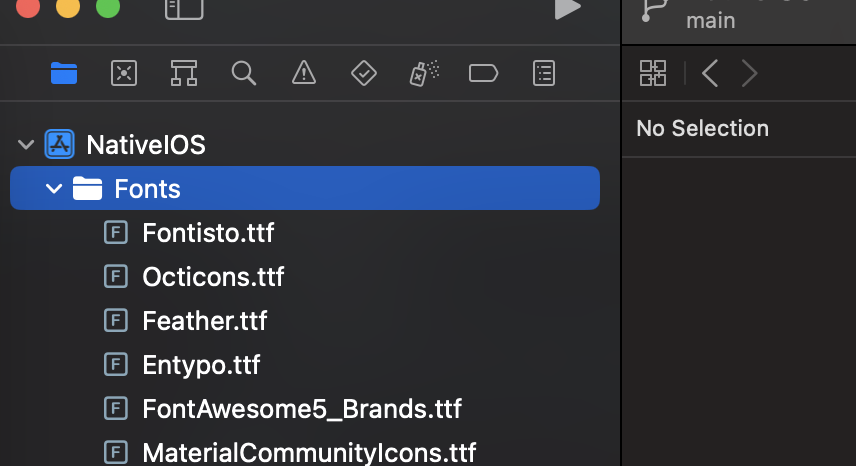
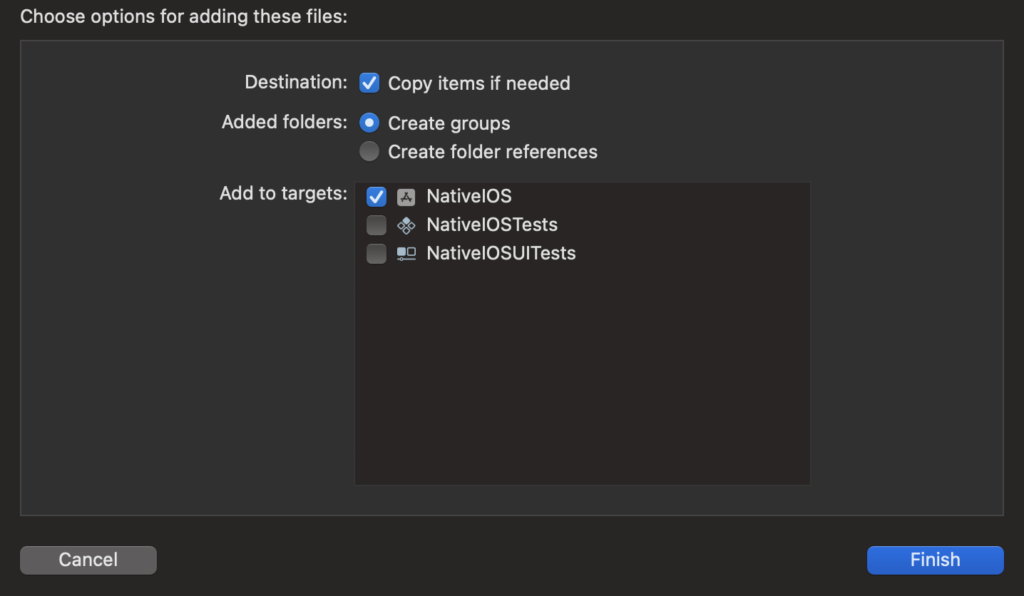
- Add this font name into info.plist file
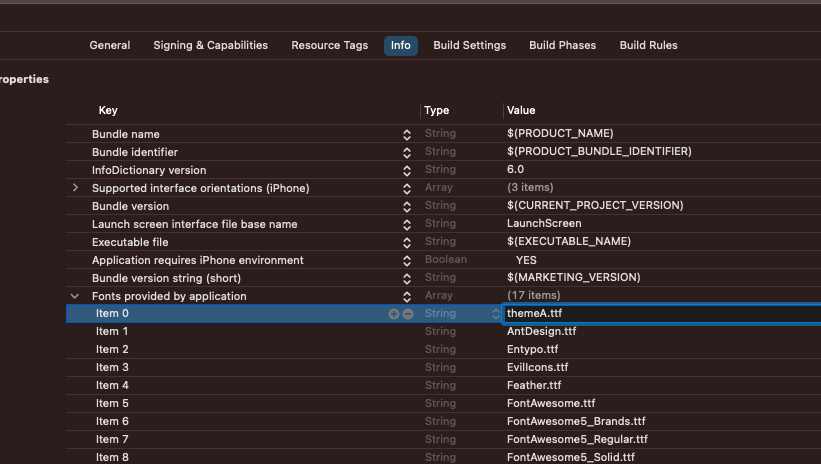
- Make sure the font file is added in Build Phases
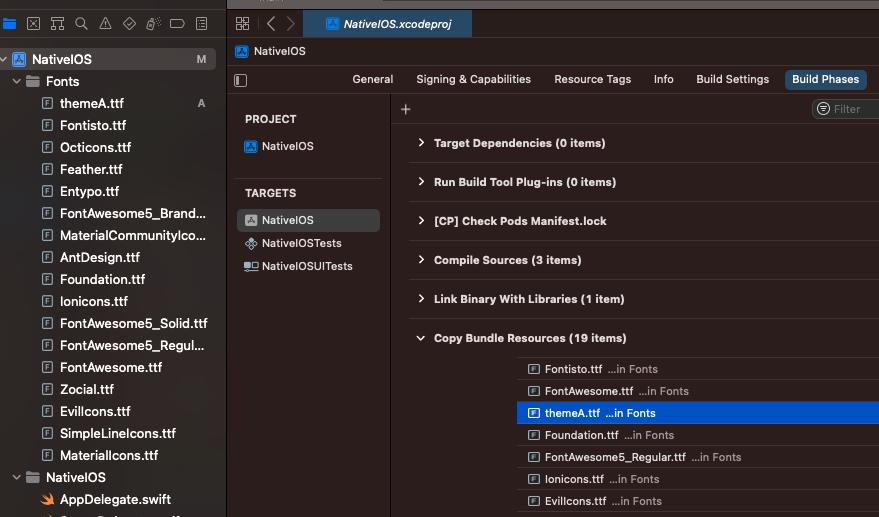
- If you see the error Unrecognized font family on iOS, it may be because the font name you are using in JS is not correct.
Remember that the Android uses name of the file but the iOS doesn’t- Add the following log to see what the font name is in ViewController file
override func viewDidLoad() { super.viewDidLoad() for family: String in UIFont.familyNames { print(family) for names: String in UIFont.fontNames(forFamilyName: family) { print("== \(names)") } } }
Leave a Reply